Introduction
Ethereum recently rolled out a wallet_addEthereumChain
RPC method that allows Ethereum applications (“dapps”) to suggest chains to be added to the user’s wallet application.
The caller must specify a chain ID and some chain metadata. The wallet application may arbitrarily refuse or accept the request. null is returned if the chain was added, and an error otherwise. Read more here EIP-3085
In most cases, an error is returned while switching to an Etherum compatible chain from a dapp connecting to a user’s MetaMask Wallet, like in the following screenshot.
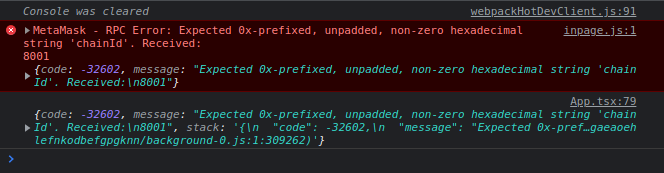
To switch to a custom RPC:
const addNetwork = () => {
const params = [{
chainId: '0x13881', // 8001
chainName: 'Mumbai',
nativeCurrency: {
name: 'MATIC Token',
symbol: 'MATIC',
decimals: 18
},
rpcUrls: ['https://matic-mumbai.chainstacklabs.com/'],
blockExplorerUrls: ['https://mumbai.polygonscan.com/']]
window.ethereum.request({ method: 'wallet_addEthereumChain', params })
.then(() => console.log('Success'))
.catch((error) => console.log("Error: " + error.message))
}
The error is thrown due to any of the following reasons:
- RPC call to
wallet_addEthereumChain
returns an error because the chain was not added. - RPC call times out leading to failure adding the custom chain.
How to resolve this error
The best way to resolve this error is:
-
Switching to another provider in
rpcUrls
property. This mostly fixes the issue as the supplied provider could be “busy”. -
Converting
chainId
fromdecimal
toHexadecimal
inPOLYGON_TESTNET_PARAMS
andPOLYGON_MAINNET_PARAMS
constants. Then add0x
prefix to change the chaindId intohexadecimal
notation. A good converter can be found here decimal-to-hex or can be done using pen and paper the old-school way!
// constants.ts
const POLYGON_TESTNET_PARAMS = {
// other properties omitted
chainId: '0x13881', // chainId in hexadecimal, decimal equivalent is 80001
// other properties ommitted
}
This is usually a problem with the provider timing out because the provider could be “busy” hence the request times out.
A complete tutorial on switching to a custom RPC can be found here
It is more straight forward to use @web3-react/injected-connector
npm package to add custom networks.